The use case I had in mind with this script is a player inventory in which an item can be viewed ‘up close’. The player would bring this view up and the camera will rotate around the item automatically at a set speed.
We are going to setup a scene where we have a ‘stage’ with a model, our item, sitting on it. We’d like the camera to rotate while facing this model the entire time.
Here’s what we’re trying to achieve:
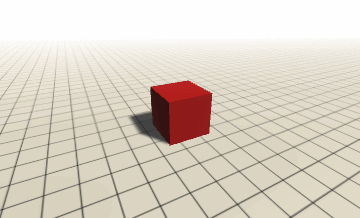
I’ve included a downloadable example at the end.
Let’s get started.
Setting up the Scene
We will need a camera and an object to target.
Here’s what my hierarchy looks like:
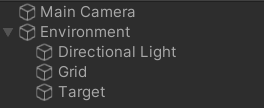
My target is just a cube.
I moved my camera so that it’s just above the target cube. This will allow us to get a view of the cube from the top as it rotates around. The starting position of the camera is up to you.
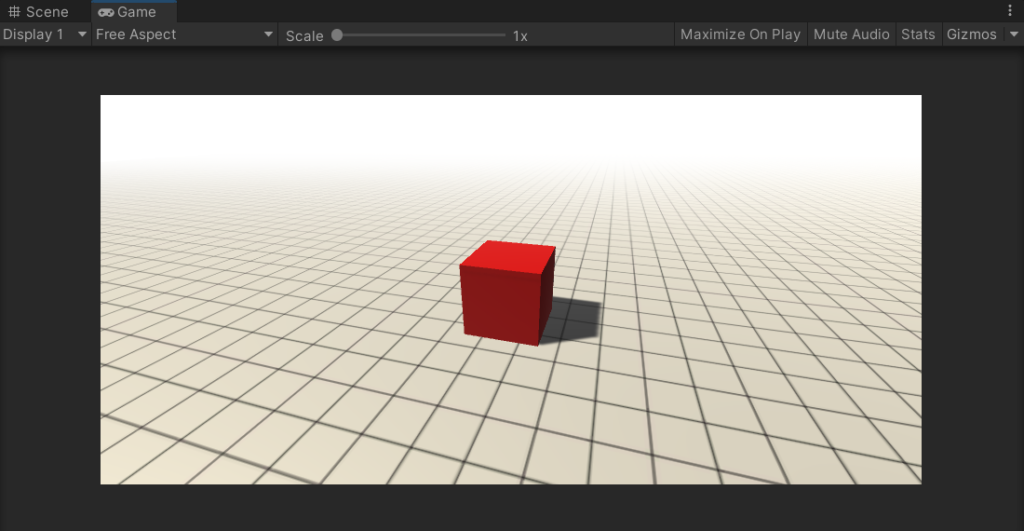
The Rotating Camera Script
You need a reference to the target’s transform component.
Transform.LookAt(target) is doing a lot of the heavy lifting here. This will adjust the rotation of the camera (or whatever object you attach this component to) to face the target object.
Transform.Translate(Vector3.right * Time.deltaTime * rotateSpeed) will affects the camera’s position and will cause it to rotate around a center point.
using UnityEngine;
public class AutoLookAndRotateCamera : MonoBehaviour
{
[SerializeField]
private Transform target;
[SerializeField]
private float rotateSpeed = 1f;
void Update()
{
transform.LookAt(target);
transform.Translate(Vector3.right * Time.deltaTime * rotateSpeed);
}
}
Now attach the AutoLookAndRotateCamera to the camera in your scene. This component requires a target so drag in a reference to what you’d like to look at (the cube in this case).
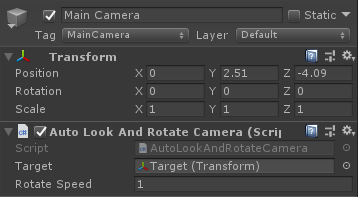
That’s it!
Want the Camera to Follow the Target?
Say your target doesn’t stay in place. This script simply looks at the target and moves the camera along it’s right axis. We need the camera to follow the target.
The easiest way to do this is by parenting the camera to the target. Like this:
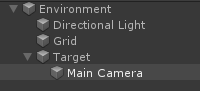
Doing it this way will cause the camera stay at a fixed distance away from the target. Now if the target moves, the camera moves along with it all the while rotating in a circle around the target.
Download the Example Project
I’ve provided a Unity package with both the scene and the camera script. Feel free to build from it.
Download: [AutoRotatingCameraExample.unitypackage]
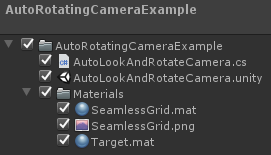